Today we’ll learn about basic input and output, but because it’s a short lesson and we’re already on day 6, I’ll also present some exercises for you to test out what you have learned up to now.
Input and output are very basic yet very important aspects of any computer program: it is what allows you to communicate with the devices attached to your computer. The most basic and simpler devices you can communicate to are the keyboard and storage devices (reading and writing files).
The idea behind input and output is to read data given by the user of your program, process it, and execute some action based on it. This gives your program the ability to work based on data on its surroundings, and to give the user control over what your application needs to do.
As said earlier, the most basic form of user interaction with a program is the keyboard. Before GUIs existed we only had command-line programs that read user input from the keyboard and based its flow of execution on it.
How one reads user input from the keyboard depends on the programming language, as they all have different internal functions to do so, but the idea is always the same: the function reads what the user inputs and stores it into a string (an array of characters) or simply read one character that you can use to determine what to do next. This pseudo-code (fake code) showcases the idea:
char input = read_input()
if input == 'c':
do something
else if input == 'a':
do something else
while input == 'b':
do something
input = read_input()
It’s not at all hard to understand this concept. You can refer to your programming language of choice to learn how these functions works and how to use them. It is also important to note that you can “reply” back to the user (output) with a text message using what is normally called the “print” function (as most languages call it “print” one way or another), but this is so basic that I won’t even get into it, a simple “Hello World” program is enough to learn how to print to screen.
Another basic and really important input/output mechanism is file reading and writing. Programs can read from and write to files to store and retrieve information, which could be text or simply binary information (text is also binary information but treated specially).
To be able to use files a program must first open it, this tells the Operating System that you are going to operate on the file, and if the file is available to operate on (i.e. no other process is using it) then the file is correctly opened and you can use it yourself. To open a file you must of course specify its path so the OS can find it, this is sort of how it works:
File my_opened_file = open_file("/path/to/file.txt")
But there’s more to it than that. You can do two basic operations to a file: read from it or write to it. Depending on the operating environment, if a file is only being read then it is safe to allow it to be opened more than once, by different processes or threads or functions, etc. But when writing to a file, it is never safe to have another code also working on that file, be it read or write. So a file can only be opened once for writing, but it may be opened more than once for reading.
Because of this, you must specify how you are going to open the file: either read, write or both. This is done at the time of opening a file, and it usually requires a string where each character represents a mode for opening the file, but it may also be a few constant values OR’ed with each other (if you don’t know what an OR operation is I suggest you read up on boolean algebra, I made some tutorials here). There’s other important modes you need to learn about, such as appending, which opens the file for writing but instead of replacing its content, it writes at the end of the file.
Once a file has been opened for either reading or writing we can proceed to do the actual reading/writing. Reading and writing to/from a file is simple: we allocate a buffer in RAM where the data will be stored, if we read from the file then the contents of the file end up in this buffer, if we write to the file then the contents of this buffer end up in the file.
It is obvious that you can’t read from the file more than what the buffer can hold and you can’t write to the file more than the buffer is holding, this is called a memory. Some higher level languages are more controlled in the way that buffers are objects and they control what their size is and how much can be read or written from/to them. We will know more about buffers when I explain pointers in the next lesson, and we’ll learn more about objects when we get to Intermediate Programming next month.
Last but not least, when you are done working on a file, you must close it to allow other threads, processes or later code to use the file, and to make sure the changes you have made to the file will be actually flushed to disk rather than kept in a cache.
Other forms of input and output exist today that are important in everyday life: mouse, touchscreen, controls (for consoles), motion and other peripherals. But at this point if you can learn the basics of programming then you have all you need to head over to Saint Google and read up on documentation about libraries that you can use to handle them, I personally will cover some of it when I talk about Game Programming in three months (need to talk about intermediate and game programming first).
Lets move on to another topic: recursion. Along with pointers, recursion is one of the hardest aspects of programming, you can understand the concept pretty easily, but most people either have a hard time “seeing” when recursion should be used and how to implement recursion at all, or some people (like me) just “see” it and use it naturally. A friend of mine from college who is an excellent programmer who knows just about everything there is to know about programming and languages has a horrible time seeing when and how to use recursion, he understands the concept perfectly, he just doesn’t “see” when a specific problem can done better with recursion, so bear with me on this, it is not a simple concept to grasp.
We know what a function is, a function is a block of code that takes in a few arguments, processes these arguments and can return a value to the caller. Very simple function being called and returned data being stored by the caller:
int function(int x){
printf("%d\n", x); // this C code prints variable x
return x;
}
int ret_value = function(2);
Nothing strange at all, but what if I tell you that the call to the function can be inside the function itself? Pretty mindblowing right? The code would end up like this:
int function(int x){
return function(x)
}
But what happens if I call this function? Well, it keeps on calling itself. When the function is called the first time it executes code that tells it to call itself again, when it calls itself it will encounter this same code telling it to call itself. And so on and so on until your computer explodes (jk, your computer won’t explode but you will run out of stack space and the program will crash). From an iterative point of view, this code has very little difference with this other code:
while (true){
}
It’s an infinite loop, a loop that keeps on doing the same thing and never ends. The only difference is that, as I said above, the recursive code will run out of stack because every time the function is called a few CPU registers will be stored on the stack (how many CPU registers are stored depends on the actual code, on the code above I predict that two registers will be stored: the PC register, which must always be stored, and the register holding the value of the variable x, we’ll talk more about this in two months when we go into badass testosterone mode and tackle low-level advanced programming).
But for the iterative while loop we could always end it, by either replacing the “true” with a condition that actually changes or by putting a “break” sentence somewhere on the code, but how do we break a recursive loop? Well that is actually quite simple: by stopping the function before it reaches the code that calls itself. And how do we stop a function’s flow of execution? Yes, the “return” statement. This effectively renders the above infinite loop useless:
int function(int x){
return x;
return function(x);
}
The first return will avoid the second return and effectively end the loop, so how can we use this to our advantage? Well, first of all lets take a look at how iterative while loops work, this is a pretty normal while loop:
while (condition){
do some code
}
The above code will become this pseudo-machine imperative code:
loop:
// we now check and evaluate the condition, and if it is not met
// we end the loop by skipping all over it
if condition not met: go to the end of the loop
execute loop // this is the actual loop's code
go to start of loop // this will make it go to the start of the loop always
loop end:
If you pay close attention, we can easily map the above into a recursive function:
int recursiveFunction(int x){
if (condition) // condition can be anything
return 0; // stops the loop
return recursiveFunction(x); // condition was not met, execute next iteration
}
Actually, the only difference between an iterative loop and a recursive function is that on each iteration of the iterative loop the variables will stay modified (except those declared on the while loop itself as they will loose their scope after each iteration), while on an iteration of a recursive function, all the variables from the previous iteration will be saved on stack and new variables (new stack space) will be used for the new ones.
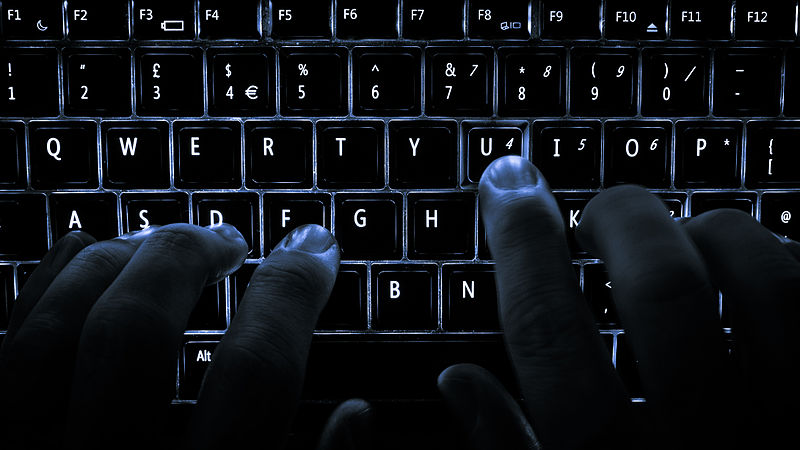
© User:Colin / Wikimedia Commons /
Now, it is important to know that you can pass any argument in the recursive call, they don’t have to be the same exact arguments that where passed onto you, for example:
int recursiveFunction(int x){
return recursiveFunction(0); // doesn't matter what I received by parameters, I can pass down whatever I want.
}
Don’t forget that a recursive call is still just a normal call, it is not treated any specially by the compiler, the same rules that applies when doing normal function calls apply here. Here’s an example that counts from whatever number you tell it to all the way up to 10:
void recursiveCount(int x){
if (x == 10)
return;
printf("%d\n", x); // show the number on screen
recursiveCount(x+1); // do the same but with an incremented x
// this will continue until x equals 10
}
In engineering terminology, the “return” statement or statements that break the recursive loop are called the base cases (there can be more than one, the same way there can be more than one reasons to break a while loop) and the recursive call is called, well, recursive case.
As with all other function calls, there can be any amount of recursive calls, there can be recursive calls that have one parameter or another depending on some condition, etc, anything that applies to regular calls also apply here. Your only concern with recursive functions is to guarantee that it will not enter an infinite loop or have too many iterations that it ends up breaking the stack.
Btw, since you guys like to do the “first” comment *** on all posts here’s a little exercise to see who is the real “first”: the above function that counts from 0 to 10 has a mayor flaw in it, can you tell what it is?
Now that we’ve covered all we needed to cover today, and before we tackle another programming heck for beginners, I’ll put up some exercises for you to test out what you have learned so far.
You can do the exercises in any programming language, but do know that I will only be able to help you with C, C++, Python, Java and to some extent C# and Perl, preferably C/C++ or Python as those are the only languages I know that I personally consider a worthwhile investment learning them.
Exercise 1:
You have to create a for loop that initializes an array of 10 elements.
If an element is in an even position then we initialize it to 0, if it’s an odd position then initialize it to 1. A number is even when the remainder of diving it by 2 is 0, an operation exists called modulo that takes the remainder of a division, search around on the web about how to do a modulo operation on your language of choice. Extra points are given if you can achieve this in just one line of code.
Exercise 2:
Create a function that given two numbers a and b, it returns the result of elevating a to the power of b and elevating b to the power of a.
If a to the power of b is bigger than b to the power of a, then it returns (a**b)/(b**a), but if (b**a) is bigger than (a**b) then it returns (b**a)/(a**b), if they are equal, it must return (a**a)/(b**b).
Extra points will be awarded to those who can optimize the code.
You cannot use a language’s built-in function to calculate the power of a number to another number, you must write your own code. Readability and usability counts.
Exercise 3:
In math, number e is an irrational number similar to pi that is used in many areas of studies. This number, often called euler’s number, can be approximated by using the following mathematical serie:
In other words, e can be approximated by doing:
The more the series continues (in other words, the bigger n is) the more approximate the number is to e.
Your job is to write a recursive function that calculates number e with the given precision n. You also need to write a separate function to calculate the factorial of a number (it may be iterative or recursive). To give you a head start, this is what the header of the function should look like:
double calculateE(int n);
Exercise for advanced programmers:
Some people complain that this is too basic stuff: duh! it’s called 10 Days of BASIC Programming, I can’t force advance stuff into newbies’ throats.
But for those of you who are bored and want to do something, here’s a challenge for ya:
Write a function that calculates a power in logarithmic time O(logn).
I have opened a thread at /talk where you can ask questions and post your answers to the above exercises, you can find it here.
<<< Previous: 10 Days of Basic Programming, Day 5: containers and declarations
>>> Next: 10 Days of Basic Programming, Day 7: Pointers
The post 10 Days of Basic Programming, Day 6: Input/Output, recursion and some exercises appeared first on Wololo.net.