One of the simplest things that can exist in any programming language: variables. Yet also the most important thing.
What are they? What are they used for? What are they made of?
Let’s say we are doing a very complicated math calculation, something like this:
2^20 * 3 + 356
It’s not something from another world, just a simple power, multiplication and addition. However doing all of that at once is overkill, so we solve the problem bit by bit, remembering the result of each operation. Let’s say in the above we take the 2^20 part aside and replace it in the equation with a significant name:
power_calc = 2^20
power_calc * 3 + 356
Now we just solve 2^20 separately and replace the value in the equation:
power_calc = 2^20 = 1048576
1048576 * 3 + 356
Now we do the same but this time with the multiplication, we isolate it, give it a name, resolve it and replace it in the equation:
mul = 1048576 * 3
mul + 356
And so on. As you can see, we use names to temporarily store values that we are going to use later on somewhere else. This is the very basic idea of what a variable is, both in math or in programming.
In programming variables are no different: they have a name to refer to them and they hold a value. It is as simple as that. But in math it’s easy, all variables always store numbers. In programming, a variable is also a number, but what that number can mean just about anything: number, memory address, letters/characters, anything. What makes programming variables so special is that we the programmers MUST define what the variable is and how it is used.
In most languages, specially C and derivatives, to declare variables you must declare what the type of the variable is. This is called strongly-typed languages. Other languages such as python (and most high-level languages) don’t need the variable type to be declared, these are called dynamically-typed languages.
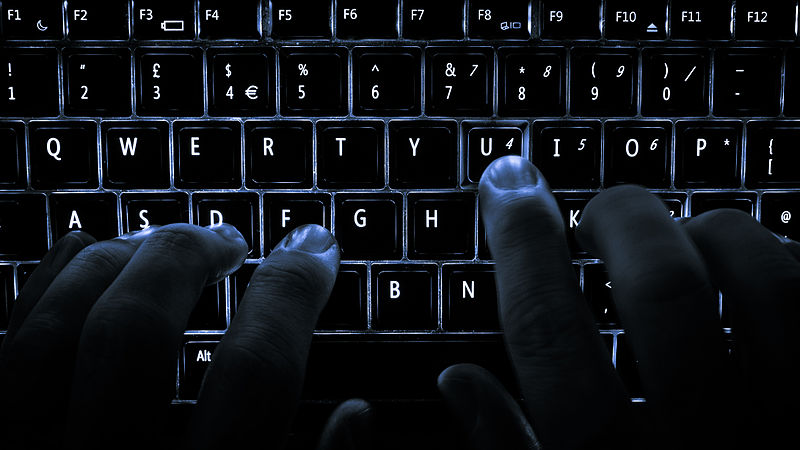
© User:Colin / Wikimedia Commons /
The reason why the variable type needs to be specified is because not all variable types take up the same space. For example a char (variable representing an ASCII character) takes up 1 byte, but a short int takes up 2 bytes, a normal int takes up 4 bytes and a long int takes up 8.
But not only space, it also tells the compiler HOW to treat the variable.
For example, an unsigned int is a number that is only positive (has no negative sign), therefore it needs to be treated differently than a normal int that can be both positive and negative.
For dynamically typed languages, variables don’t need to have their type declared because technically speaking all variables are of the same type: pointers. Therefore they all have the same size and the same way to use them, so there’s no need to declare them. Type does need to be known but the compiler generates it automatically. We’ll see more about this when we get to pointers and even more when we get to Object Oriented Programming (next month).
Now there is one very important thing to know for strong-typed languages: there is a HUGE difference between declaring the variable and initializing it. Declaring the variable is you telling the compiler that a variable named X of type Y will eventually be used, but not now. Initializing it is ACTUALLY using the variable. In a C like code, this is a declared variable:
int x
We are telling the compiler that at one point we’ll be using a variable named x of type int, so the compiler allocates memory for this variable for when we are going to use it. We’ll see more about this when I talk about the stack and data segments. However, initializing the variable is actually using it:
x = 0
Here we actually use the variable x by assigning the value 0 to it.
However, any experienced programmer will tell you that you should declare and initialize the variable at the same time, which can be done like this:
int x = 0
Here we tell the compiler that we’re going to use a variable named x of type int and initial value of 0.
In dynamic languages like python we don’t need to declare the type of the variable as the type is implicit, we just initialize the variable to tell the interpreter that a new variable is going to be used:
x = 0
Other languages that are dynamically typed use a combination of both: variables don’t have an explicit type, but to declare them you have to use some sort of keyword, usually “var”, like in JavaScript:
var x = 0
Variables are used to store values that we are going to temporarily use (stack variables), or that are globally available (data-segment variables).
What determines if a variable is local or global is what we call the scope of the variable, but that’s something we’ll learn in the next session: functions.
Here are some of the most common and basic variable types in C and derivatives:
– int: standard integer type.
– short: an integer type that is half the size of int
– long: an integer type that is double the size of int
– char: a variable that represents a character (letters, numbers, symbols, etc), it takes up 1 byte in C/C++ and 2 bytes in Java. This is due to the fact that C/C++ char uses the ASCII character table while Java’s uses Unicode.
– unsigned: this is a type modifier that must be accompanied by other types such as int, short, long and char. This tells the compiler that the specified type doesn’t have a sign. A normal integer uses it’s first bit to indicate sign, 0 means positive, 1 means negative, but this modifier makes the CPU treat that bit not as the sign but as part of the number itself. For example, a signed char can take values from -128 to 127, but an unsigned char can take values from o to 255. It is worth noting that Java doesn’t have this type modifier, this is one of the reasons Java has been criticized. Say you have a number that is between 128 and 255, in C this number can be represented with 1 byte by using unsigned char, but in Java you have to use a short, which requires you to do unnecessary castings and take up 2 bytes instead.
– float: float is a standard type defined by IEEE, it is used to represent decimal numbers: 1.6, 2.8, 167.396, etc. Unlike integers, floats can’t be unsigned.
– double: double is the same as float but it takes up double t
<<< Previous: 10 Days of Basic Programming, Day 1: Introduction
>>> Next: 10 Days of Programming, Day 3: Flow Control
The post 10 Days of Basic Programming, Day 2: Variables appeared first on Wololo.net.