One of the things that define a computer is its ability to do something or the other depending on certain conditions.
Today I will teach you how to declare these conditions and the code that will be executed when the conditions are met or when the conditions are not met.
The reason programming languages are called “languages” is because they utilize constructs similar to human language. Let’s say you ask a friend to go get you something to eat at the supermarket. You ask them to bring you orange juice, and if there’s no orange juice, to bring you apple juice.
The above example can be easily mapped in a programming term like this:
if there's orange juice:
bring back orange juice
else:
bring back apple juice
But what if there’s no apple juice either? Then let’s say you tell your friend to bring back any other random drink, like this:
if there's orange juice:
bring back orange juice
else if there's apple juice:
bring back apple juice
else:
bring back anything else
This in programming terms is what is called flow control. We tell the system to execute certain tasks depending on certain conditions.
There are many different flow control statements that achieve different functionality, but the most common are the if-else if-else statements as shown above, but there is also loops.
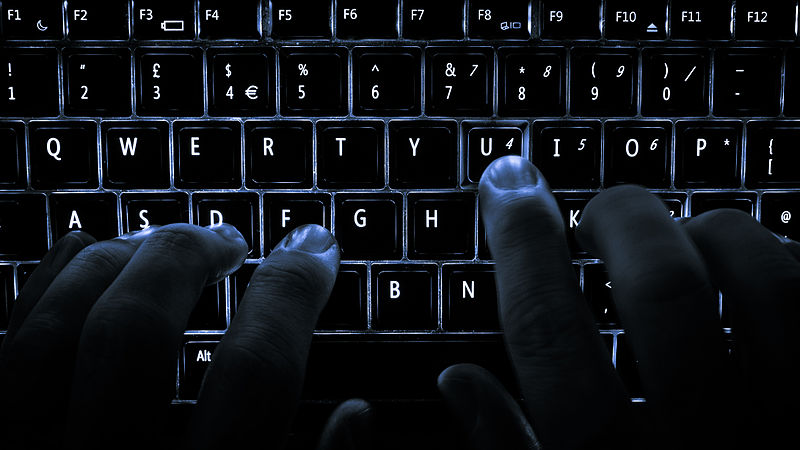
© User:Colin / Wikimedia Commons /
A loop is something that happens repeatedly. Let’s say that you tell your friend that if there’s no orange juice to wait until it is replenished.
In programming terminology, this can be expressed the following way:
while there's no orange juice:
wait for orange juice to be replenished
bring back orange juice once it's replenished
But… what if orange juice is never replenished? Your friend will be waiting forever! This is what we call an infinite or endless loop and is something we never want to happen, so you have to tell your friend to only wait for only half an hour, and if they haven’t replenished it in that time, to bring you back the apple juice:
while there's no orange juice:
wait for orange juice to be replenished
if half an hour has passed:
bring back apple juice
if orange juice replenished:
bring back orange juice
Now let’s take into consideration the following: when you just arrive at the supermarket and no orange juice is there, it makes no sense to start checking if half an hour has passed. This is a special condition that prevents the loop code from being executed, so we must skip it and continue with checking the loop’s condition:
while there's no orange juice:
wait for orange juice to be replenished
if I just arrived:
continue checking loop
if half an hour has passed:
bring back apple juice
if orange juice replenished:
bring back orange juice
This creates a special keyword called “continue”, which basically means that, skip the loop’s code and continue with the loop.
But now, what if orange juice just arrives exactly after you checked the loop’s condition and it is about to pass half an hour? We’re in trouble as we’d bring back apple juice even though they just replenished orange juice.
So there must be a way to immediately stop the loop and prevent it from continue executing. This is the “break” statement.
A loop construct can be represented as an if that keeps executing until the if becomes false:
if there's no orange juice:
wait for orange juice
check for orange juice again by doing the above if
In programming terminology the above “commands” if and while are called statements. These two statements require checking that a certain condition is met to execute it’s code. The condition is evaluated to what we call a boolean value, which means it is either true or false. As you ay have guessed, if the statement evaluates to true, the code is executed, if it evaluates to false it isn’t, and in the case of if, the code in the else is executed.
This is the syntax for the if and while statements in C and C-like languages:
if (condition){
execute this code
}
else {
execute this other code
}
while (condition){
execute this as long as condition is still true
}
On the subject of loops, there are three more (important) loops; do-while, for and foreach.
Do-while is technically the same as a while, but the condition for the while is evaluated AFTER the code is executed, this means that the code is guaranteed to be executed at least once. It’s C syntax is like this:
do {
execute code
} while (condition);
For loops are a little harder to understand at first, but don’t worry we’ll see them more often so you’ll get the idea behind them eventually.
A for loop is the same as a while, but it has three parts: initialization, condition evaluation and condition update. In other words, the for loop does this:
– initialize a variable
– check a condition <– this is the same as a while loop
– execute code if condition is true <– same as while
– update the variable
The simplest way to understand a for loop is with counters.
Let’s say you want to count from 0 to 10, you can do it with a while loop like this:
int counter = 0;
while (counter < 11){
counter = counter + 1;
}
But of course there’s an easier way to do it: for loops. The above can be translated into the following for loop:
for (int counter=0; counter<11; counter=counter+1){
}
As you can see, for loops are generally used when we want to execute a piece of code an amount of times that is known, but the while loop is used when we don’t know exactly when we are going to stop executing the code.
Technically, we can use for loops just like while loops but omitting the initialization and update of the variable, like this:
for (;condition;){
}
Finally, there’s foreach loops. Foreach loops are not something that existed in C as is, it is normally found on higher level languages.
A foreach loop is used when we want to work with collections (we’ll look into that later). Basically this is the overall construct of a foreach loop:
foreach item in list:
do something with item
Foreach are hard to understand at this point, so if you don’t get the overall idea behind them, don’t worry, we’ll look at them when we get to high-level programming.
To finish off flow control statements, we’re gonna talk about another famous statement: the switch statement.
To better understand the switch statement, let’s just look at what a real-life switch does: when the switch is in the off state, the lights are off, if the switch is on, the lights are on. In a programming fashion:
switch:
ON: lights are on
OFF: lights are off
In programming, switchs require, much like if and while, a variable to be evaluated by comparing with the values and as how many values that need to be taken into consideration to execute something or the other, like this:
switch variable:
value 1: do something
value 2: do something
value 3: do something
Switch statements compare the variable with the value given and if equal that code is executed. Last but not least, switch statements allow to have a “default” statement that is executed when the variable isn’t equal to any of the values.
Now, you may think that there’s no difference between switch and if-else other than how they work, and for the most part you’re right, except that: switch statement is a lot more readable than if-else and on certain occasions the compiler can optimize them to be much more faster than a bunch of if-else.
In C, this is the syntax for switch statements:
switch (variable){
case value1: break;
case value2: break;
case value3: break;
default: break;
}
Notice the “break” in all of them, if you do not a break statement at the end of each case then the case following it will be executed, which is generally not desired.
Also note that the values MUST be constant and they can only be integers (and by extension, characters too as they are really just integers).
As an extra note: Python doesn’t have normal for loops, only foreach, and doesn’t have switch. Python also has the “elif” statement, which is nothing but a contraction of “else if”.
<<< Previous: 10 Days of Basic Programming, Day 2: Variables
>>>Next: 10 Days of Basic Programming, Day 4: functions and scope
The post 10 Days of Programming, Day 3: Flow Control appeared first on Wololo.net.